Last post
Copy bitmap using getPixels() and setPixels() show how to copy a whole Bitmap using getPixels() and setPixels(). It can be used to copy part of a Bitmap.
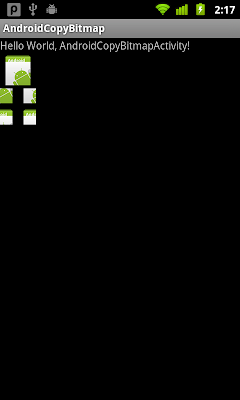
package com.AndroidCopyBitmap;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Matrix;
import android.os.Bundle;
import android.widget.ImageView;
public class AndroidCopyBitmapActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ImageView image1 = (ImageView)findViewById(R.id.image1);
ImageView image2 = (ImageView)findViewById(R.id.image2);
Bitmap oldBitmap = BitmapFactory.decodeResource(getResources(), R.drawable.ic_launcher);
int orgWidth = oldBitmap.getWidth();
int orgHeight = oldBitmap.getHeight();
int firstx, firsty;
int halfWidth = orgWidth/2;
int halfHeight = orgHeight/2;
//Create a same size Bitmap
Bitmap newBitmap = Bitmap.createBitmap(orgWidth, orgHeight, Bitmap.Config.ARGB_8888);
//Create a pixel of 1/4 size, to copy part of the bitmap
int[] pixels = new int[halfWidth * halfHeight];
//Copy Upper-Left part to Bottom-Right
oldBitmap.getPixels(pixels, 0, halfWidth, 0, 0, halfWidth, halfHeight);
newBitmap.setPixels(pixels, 0, halfWidth, halfWidth, halfHeight, halfWidth, halfHeight);
//Copy Bottom-Right to Upper-Left part
oldBitmap.getPixels(pixels, 0, halfWidth, halfWidth, halfHeight, halfWidth, halfHeight);
newBitmap.setPixels(pixels, 0, halfWidth, 0, 0, halfWidth, halfHeight);
//Copy Bottom-Left part to Upper-Right
oldBitmap.getPixels(pixels, 0, halfWidth, 0, halfHeight, halfWidth, halfHeight);
newBitmap.setPixels(pixels, 0, halfWidth, halfWidth, 0, halfWidth, halfHeight);
//Copy Upper-Right to Bottom-Left part
oldBitmap.getPixels(pixels, 0, halfWidth, halfWidth, 0, halfWidth, halfHeight);
newBitmap.setPixels(pixels, 0, halfWidth, 0, halfHeight, halfWidth, halfHeight);
image1.setImageBitmap(oldBitmap);
image2.setImageBitmap(newBitmap);
}
}
No comments:
Post a Comment